Let’s discuss the question: how to iterate through a singly linked list. We summarize all relevant answers in section Q&A of website Achievetampabay.org in category: Blog Finance. See more related questions in the comments below.
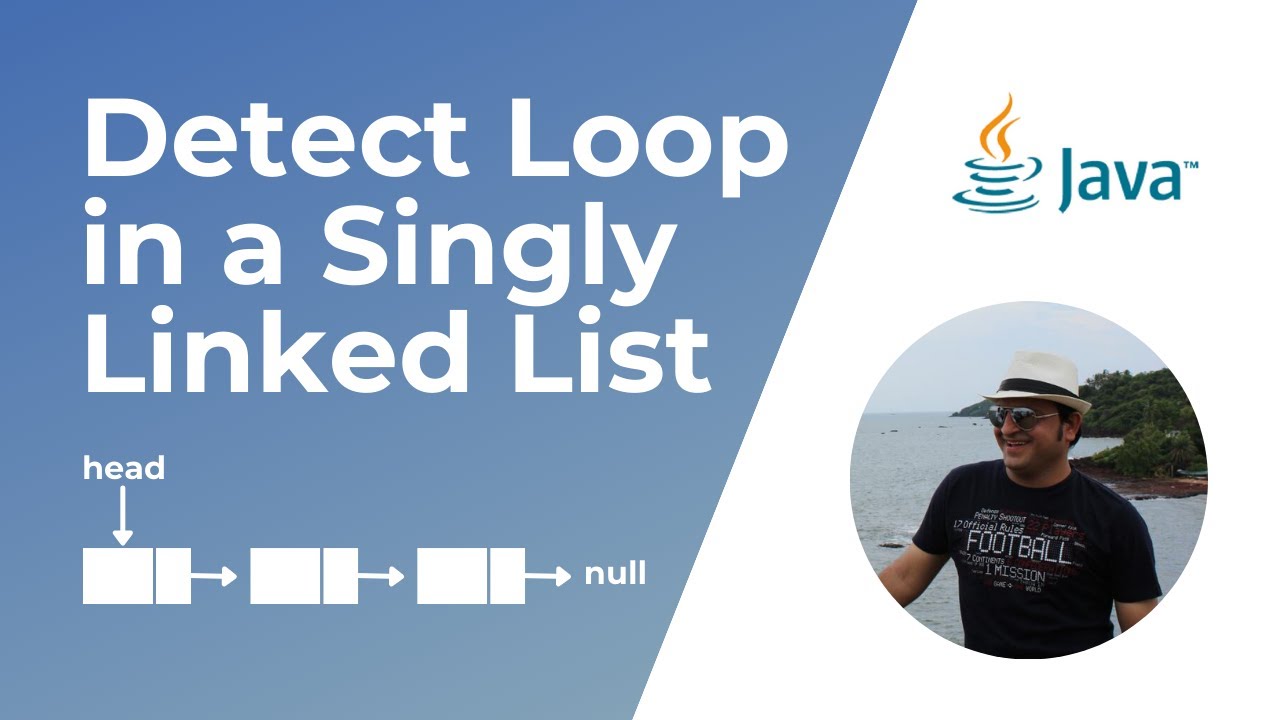
How do you iterate through a linked list in Python?
In order to traverse a linked list, you just need to know the memory location or reference of the first node, the rest of nodes can be sequentially traversed using the reference to the next element in each node. The reference to the first node is also known as the start node.
How do you make a loop in a linked list?
- Initialize a count variable with 1 and a variable temp with the first node of the list.
- Run a while loop till the count is less than K. …
- Save the temp in the kth_node variable.
- Run a while loop till temp is not NULL. …
- At last, connect temp with kth_node i.e., temp->next = kth_node.
How to detect a loop in a Singly Linked List in Java ?
Images related to the topicHow to detect a loop in a Singly Linked List in Java ?
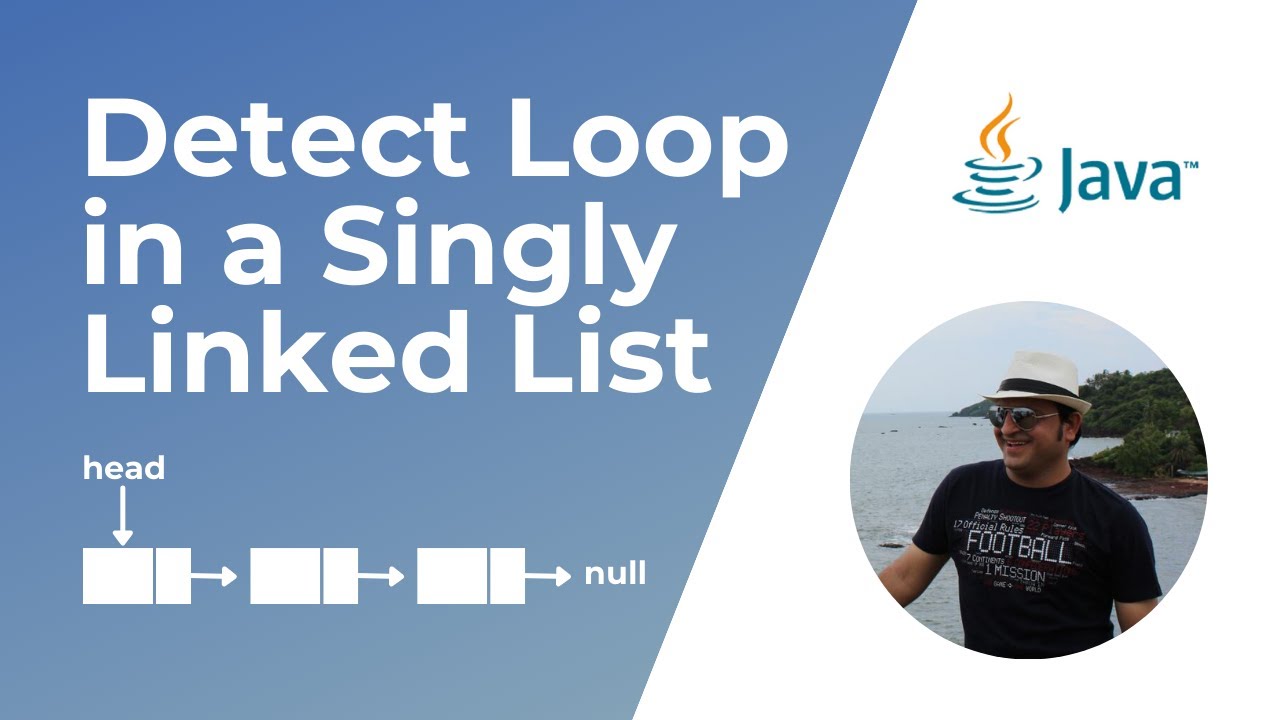
How do you traverse a singly linked list in C#?
For a singly-linked list, only forward direction traversal is possible. Using InsertNext() insert the elements into the list. In traverse() function, if condition statement is used to check that the value of ‘node’ variable is equal to null. If the condition is true then execute the statement.
How do you cycle through a linked list in C++?
Traversing through a linked list is very easy. It requires creating a temp node pointing to the head of the list. If the temp node is not null, display its content and move to the next node using temp next. Repeat the process till the temp node becomes null.
What is singly linked list?
A singly linked list is a type of linked list that is unidirectional, that is, it can be traversed in only one direction from head to the last node (tail). Each element in a linked list is called a node. A single node contains data and a pointer to the next node which helps in maintaining the structure of the list.
How do you create a singly linked list in Python?
- # A single node of a singly linked list.
- class Node:
- # constructor.
- def __init__(self, data = None, next=None):
- self. data = data.
- self. next = next.
- # A Linked List class with a single head node.
How do you know if a singly linked list is cyclic?
- 1) Use two pointers fast and slow.
- 2) Move fast two nodes and slow one node in each iteration.
- 3) If fast and slow meet then the linked list contains a cycle.
- 4) if fast points to null or fast.next points to null then linked list is not cyclic.
Does linked list have loop?
A linked list contains a cycle if it consists of a node that can be reached again by continuously following the next pointer. Explanation: The linked list consists of a loop, where the last node connects to the second node.
Can you use for loop in linked list?
Using enhanced for loop we can sequentially iterate a LinkedList. The execution of the enhanced for loop ends after we visit all the elements. Let us see an example of iterating the LinkedList using the enhanced for loop.
Which of the following loops could be used to iterate through an entire LinkedList?
Foreach The easiest way to loop through and examine all the contents of a LinkedList is with the foreach-loop. Find, insert. We pass an element value to Find.
What is LinkedListNode C#?
Each element of the LinkedList<T> collection is a LinkedListNode<T>. The LinkedListNode<T> contains a value, a reference to the LinkedList<T> that it belongs to, a reference to the next node, and a reference to the previous node.
Traversing a Single Linked List (Counting the Nodes)
Images related to the topicTraversing a Single Linked List (Counting the Nodes)
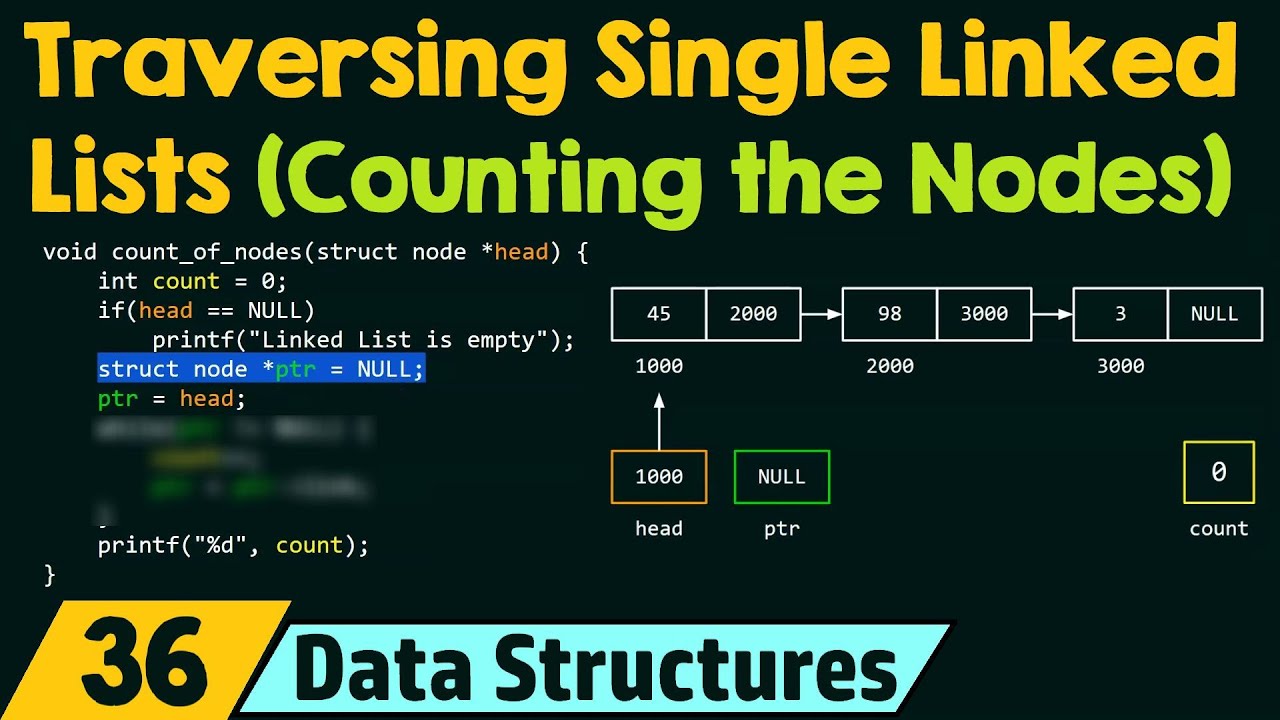
How do you traverse a linked list from tail?
- Initialize three pointers prev as NULL, curr as head and next as NULL.
- Iterate through the linked list. In loop, do following. // Before changing next of current, // store next node. next = curr->next. // Now change next of current. // This is where actual reversing happens. curr->next = prev.
What is a cycle in a linked list?
A cycle occurs when a node’s next points back to a previous node in the list. The linked list is no longer linear with a beginning and end—instead, it cycles through a loop of nodes.
How do you insert a node at the beginning of a linked list C++?
- A function insertAthead(node*&head, int data) takes the address of the head node and the data which we have to insert.
- Create a new node and insert the data into it.
- Move the head to the newly created node.
- Print the linked list.
What are the parts of a singly linked list node?
A linked list consists of items called “Nodes” which contain two parts. The first part stores the actual data and the second part has a pointer that points to the next node. This structure is usually called “Singly linked list”.
What is singly and doubly linked list?
A Singly Linked has nodes with a data field and a next link field. A Doubly Linked List has a previous link field along with a data field and a next link field. In a Singly Linked List, the traversal can only be done using the link of the next node.
What is a singly linked list Java?
The singly linked list is a linear data structure in which each element of the list contains a pointer which points to the next element in the list. Each element in the singly linked list is called a node.
What is a singly linked list in Python?
Singly linked lists can be traversed in only forward direction starting form the first data element. We simply print the value of the next data element by assigning the pointer of the next node to the current data element.
How do you implement a singly linked list in C++?
This is given as follows. struct Node { int data; struct Node *next; }; The function insert() inserts the data into the beginning of the linked list. It creates a new_node and inserts the number in the data field of the new_node.
What are the steps for inserting a new element into a singly linked list at its head?
- Insert at the beginning. Allocate memory for new node. Store data. Change next of new node to point to head. …
- Insert at the End. Allocate memory for new node. Store data. Traverse to last node. …
- Insert at the Middle.
Why is doubly linked list better than singly linked list?
If we need better performance while searching and memory is not a limitation in this case doubly linked list is more preferred. As singly linked list store pointer of only one node so consumes lesser memory. On other hand Doubly linked list uses more memory per node(two pointers).
Traverse a Linked List Iteratively in Java | Linked List Algorithms
Images related to the topicTraverse a Linked List Iteratively in Java | Linked List Algorithms
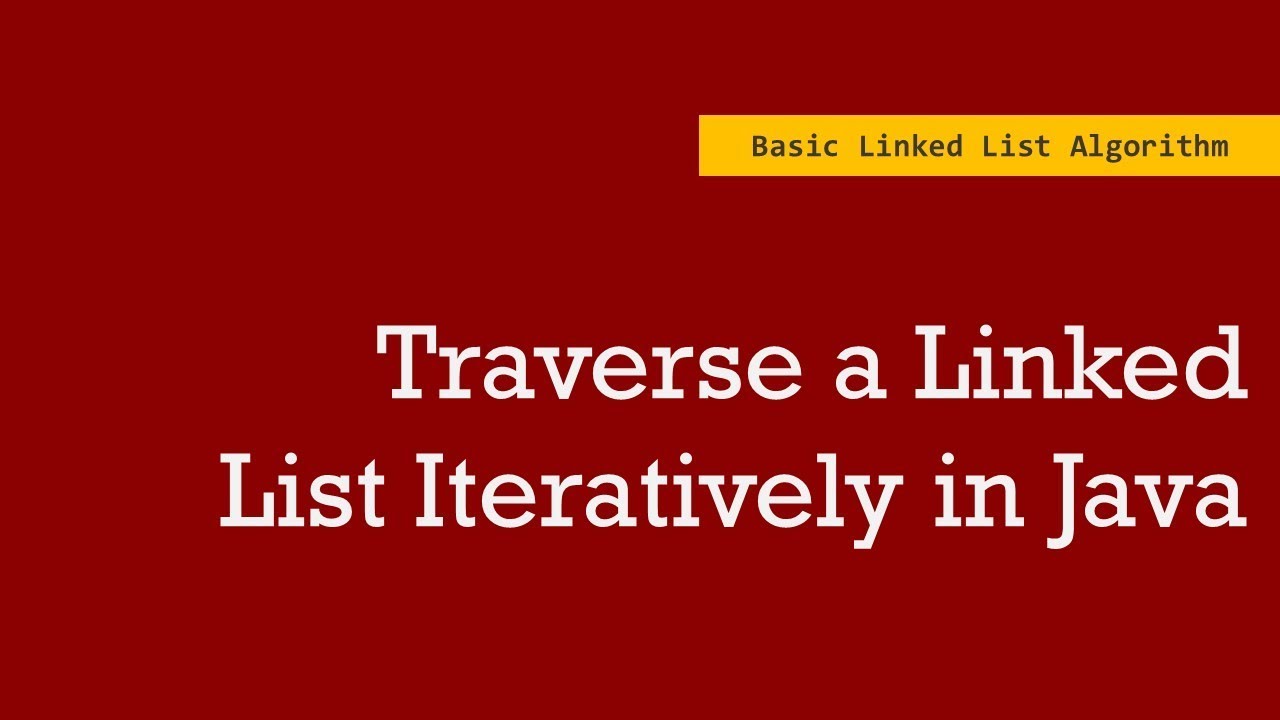
How many address fields are there in singly circular linked list?
Expert-verified answer
Only one addition reference labelled ‘Next’ exists in a single circular list.
How do you check if a doubly linked list is empty?
Let head and tail be special nodes (called “sentinel nodes”, as pointed out in the other answer) which don’t actually contain any data and point to the first and last nodes in the list through next and prev respectively. In this case, header. next == tail will mean the list is empty.
Related searches
- how to iterate through a singly linked list c++
- Singly linked list Java
- what is singly linked list explain with example
- how to iterate a linked list
- how to iterate through a linked list in c++
- loop in singly linked list
- Create a singly linked list
- how to iterate through a singly linked list python
- cycle in singly linked list
- singly linked list iterator java
- insertion in singly linked list at the end
- delete linked list c
- create a singly linked list
- how to iterate through a singly linked list java
- going through a linked list
- singly linked list java
- traverse through linked list
- how to iterate through a singly linked list c
- python linked list
Information related to the topic how to iterate through a singly linked list
Here are the search results of the thread how to iterate through a singly linked list from Bing. You can read more if you want.
You have just come across an article on the topic how to iterate through a singly linked list. If you found this article useful, please share it. Thank you very much.